sass基础
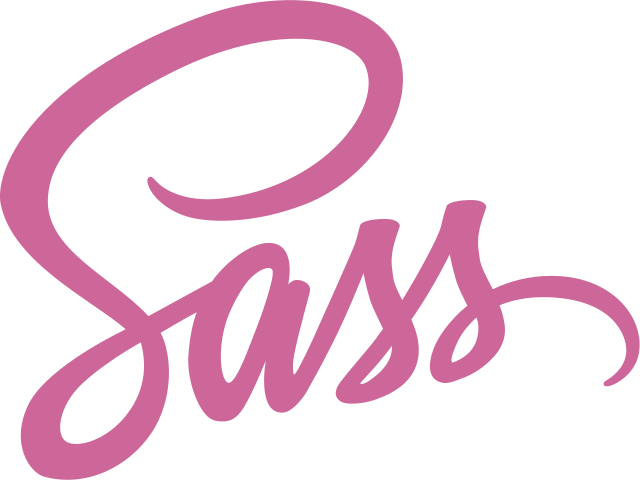
安装
全局安装 sass
npm install sass -g
新建目录
mkdir sass_learn && cd $_
目录结构
sass_learn
- sass
- style.scss
- css
- style.css
编译
sass sass/style.scss:css/style.css
监听文件变化
sass --watch sass:css
编译格式
- expanded 扩展
- compressed 压缩
sass --watch sass:css --style compressed
嵌套
body{
font:{
family: Microsoft yahei;
size: 15px;
weight: 600;
}
.nav{
border: 1px solid #000 {
left:0;
right:0;
};
}
}
mixin
@mixin alert($text-color,$background-color) {
color:$text-color;
background-color: $background-color;
a{
color:darken($text-color, 50%);
}
}
.alert-warning {
@include alert(#666,#fff);
}
.alert-info {
@include alert($background-color: #000, $text-color:#ff0)
}
extend
.alert {
padding: 15px;
}
.alert a{
font-weight: 500;
}
.alert-info {
@extend .alert;
background-color: #00ff00;
}
@import
新建文件 _base.scss
,下划线开头的文件名不会被sass编译,通过@import
引入的文件可以不用加下划线。
@import "./base.scss"
Comment
/*
* 这种注释编译后会被保存
*
*/
// 单行注释 这种注释编译后不会被保存
/*! 这种加!的注释压缩编译也会被保存 */
Data Type
$ sass -i
>> type-of(5)
number
>> type-of(5px)
number
>> type-of(hello)
string
>> type-of("hello")
string
>> type-of(1px solid #ddd)
list
>> type-of(1px 10pxd)
list
>> type-of(#00ff00)
color
>> type-of(red)
color
>> type-of(rgb(255,0,0))
color
>> type-of(hsl(0,100%,50%))
color
number
$ sass -i
>> 2+8
10
>> 2*8
16
>> 8/2
8/2
>> (8/2)
4
>> (5px+2px)
7px
>> (5px*2px)
10px*px
>> (10px/2px)
5
>> (10px/2em)
5px/em
>> (10px/2)
5px
数字函数
$ sass -i
>> abs(10)
10
>> abs(-10)
10
>> abs(-10px)
10px
>> round(3,6)
^^^^^^^^^^
Error: Only 1 argument allowed, but 2 were passed.
>> round(3.6)
4
>> round(3.6px)
4px
>> ceil(3.5)
4
>> floor(3.5)
3
>> percentage(500px/1000px)
50%
>> min(1,2,3,)
1
>> max(1,2,3)
max(1, 2, 3)
string
$ sass -i
>> "chen"+weilong
"chenweilong"
>> chen+" weilong"
chen weilong
>> 'cc'+ weilong
"ccweilong"
>> 'cc'+" weilong"
"cc weilong"
>> chen+ 99
chen99
>> chen-weilong
chen-weilong
>> chen/weilong
chen/weilong
字符串函数
$ sass -i
>> $greeting: "Chen"
"Chen"
>> to-upper-case($greeting)
"CHEN"
>> to-lower-case($greeting)
"chen"
>> str-length($greeting)
4
>> str-index($greeting,'en')
3
>> str-insert($greeting,' weilong',4)
"Che weilongn"
color
HSL
色相、饱和度、明度
.black{
background-color: rgb(0,0,0);
}
.blackOpacity{
background-color: rgba(0,0,0,.5);
}
.hsl{
background-color: hsl(50,100%,50%);
}
.hslOpacity{
background-color: hsl(50,100%,50%,.5);
}
$base-color-hsl:hsl(50,100%,50%,.5);
$light: lighten($base-color-hsl, 10%);
$darken: darken($base-color-hsl, 20%);
$saturate: saturate($base-color-hsl, 50%); // 增加饱和度
$desaturate: desaturate($base-color-hsl, 50%); // 增加饱和度
$opacify: opacify(#ff0000, 0.1);
$transparentize: transparentize(#000, .4);
.hsl{
background-color: adjust-hue($base-color-hsl, 137deg);
}
.light{
// background-color: lighten($base-color-hsl, 10%);
background-color: $light;
}
.darken{
// background-color: darken($base-color-hsl, 20%);
background-color: $darken;
}
.saturate{
background-color: $saturate
}
.desaturate{
background-color: $desaturate
}
.opacity{
background-color: $opacify;
}
.transparentize{
background-color: $transparentize
}
list
$ sass -i
>> length(5px 10px)
2
>> length(5px 10px 10px 5px)
4
>> nth(1px 2px 3px,1)
1px
>> nth(1px 2px 3px,2)
2px
>> index(1px solid #ff0,solid)
2
>> append(5px 10px,10px)
5px 10px 10px
>> join(10px 20px,30px 40px)
10px 20px 30px 40px
>> join(10px 20px,30px 40px,comma)
10px, 20px, 30px, 40px
map
$map:(key1: value, key2: value2, key3: value3)
$ sass -i
>> $colors:(light:#fff, dark:#000)
(light: #fff, dark: #000)
>> length($colors)
2
>> map-get($colors,light)
#fff
>> map-get($colors,dark)
#000
>> map-keys($colors)
light, dark
>> map-values($colors)
#fff, #000
>> map-has-key($colors,lighs)
false
>> map-has-key($colors,ligth)
false
>> map-has-key($colors,light)
true
>> map-merge($colors,(light-gray:#e5e5e5))
(light: #fff, dark: #000, light-gray: #e5e5e5)
>> $colors: map-merge($colors,(light-gray:#e5e5e5))
(light: #fff, dark: #000, light-gray: #e5e5e5)
>> $colors
(light: #fff, dark: #000, light-gray: #e5e5e5)
>> map-remove($colors,light,dark)
(light-gray: #e5e5e5)
Boolean
$ sass -i
>> 5px > 3px
true
>> 5px > 10px
false
>> (5px>10px) and (5px >4px)
false
>> (5px>2px) and (5px >4px)
true
>> (5px>2px) or (5px >4px)
true
>> (5px>2px) or (5px >14px)
true
>> not(5px>4px)
false
>> not(5px>10px)
true
Interpolation
$version: "0.0.1";
/* 项目当前版本号是:#{$version} */
$name: "info";
$attr: "border";
.alter-#{name}{
background-color: #000;
}
输出:
/* 项目当前版本号是:0.0.1 */
.alter-name {
background-color: #000;
}
控制指令 Control Directives
@if
判断
$use-prefixes: true;
.rounded{
@if $use-prefixes {
-webkit-border-radius: 5px
}
border-radius: 5px
}
输出:
.rounded {
-webkit-border-radius: 5px;
border-radius: 5px;
}
其它场景:
$theme:light;
body{
@if $theme == dark {
background-color: #000;
} @else if $theme == light {
background-color: #fff;
}
}
// 输出
body {
background-color: #fff;
}
@for
循环
$columns: 2;
// through|to 结束值为2 | 结束值为1
@for $i from 1 through $columns {
.col-#{$i} {
width: 100% / $columns * $i;
}
}
输出:
.col-1 {
width: 50%;
}
.col-2 {
width: 100%;
}
@each
遍历列表list
雪碧图
$icons: success error warning;
@each $icon in $icons {
$lenght: length($icons);
$index: index($icons,$icon);
.icon-#{$icon}{
background-image: url(./images/#{$icon}.png) 0 #{$lenght * $index}px;
}
}
输出
.icon-success {
background-image: url(./images/success.png) 0 3px;
}
.icon-error {
background-image: url(./images/error.png) 0 6px;
}
.icon-warning {
background-image: url(./images/warning.png) 0 9px;
}
@while
循环
$i: 6;
@while $i > 0{
.item-#{$i}{
width: 5px * $i;
}
$i: $i - 2;
}
function
$colors: (light: #fff, dark: #000);
@function color($key){
@return map-get($colors,$key);
}
body{
background-color: color(dark)
}
警告与错误
$colors: (light: #fff, dark: #000);
@function color($key){
@if not map-has-key($colors,$key){
@warn "在 $colors 里没有找到 #{$key} 这个 key"
}
@return map-get($colors,$key);
}
body{
background-color: color(abc)
}